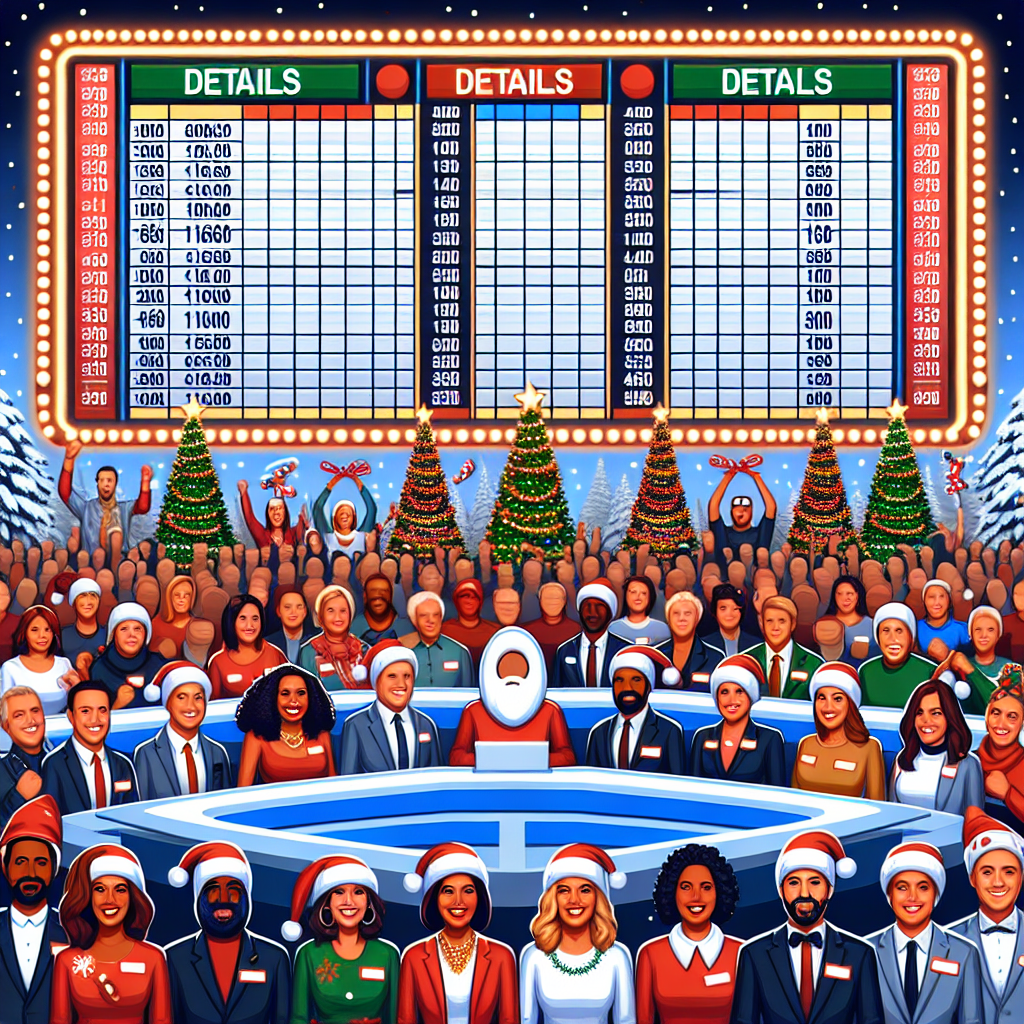
What contestant has won the most number of challenges? Select their row from the castaway_details DataFrame and save this as Q11. This should return a DataFrame and the index and missing values should be left as is.
To find the contestant who has won the most number of challenges, we need to calculate the sum of challenges won for each contestant and then select the row with the highest sum.
Here's how you can do it:
``` python
# Calculate the sum of challenges won for each contestant
challenges_won = castaway_details[['Name', 'Individual Challenges Won', 'Team Challenges Won', 'Total Challenges Won']]
challenges_won['Total Challenges Won'] = challenges_won['Individual Challenges Won'] + challenges_won['Team Challenges Won']
# Select the row with the highest sum of challenges won
Q11 = challenges_won.loc[challenges_won['Total Challenges Won'].idxmax()]
# Print the result
print(Q11)
```
This will print the row of the contestant who has won the most number of challenges. Note that `castaway_details` is the name of the DataFrame containing all the details of the castaways.
To find the contestant who has won the most number of challenges, you can use the following steps:
1. First, sort the "challenges_won" column in descending order using the `sort_values()` method.
2. To select the row with the highest value in the "challenges_won" column, you can use the `head(1)` method.
3. Finally, save this row as a DataFrame named "Q11".
Here is the code to accomplish this:
```python
Q11 = castaway_details.sort_values('challenges_won', ascending=False).head(1)
```
This will return a DataFrame with the row corresponding to the contestant who has won the most number of challenges. The index and missing values will remain unchanged.
To find the contestant who has won the most number of challenges, you can follow these steps:
1. Access the `castaway_details` DataFrame.
2. Examine the columns in the DataFrame to identify the column that contains information about the number of challenges won by each contestant.
3. Once you have identified the column, sort the DataFrame in descending order based on this column.
4. Select the row that corresponds to the contestant who has won the most number of challenges.
Here is the code to accomplish this:
```python
# Step 1: Access the castaway_details DataFrame
Q11 = castaway_details
# Step 2: Identify the column with the number of challenges won
# You will need to replace 'challenges_won' with the actual column name in your DataFrame
challenges_won_column = 'challenges_won'
# Step 3: Sort the DataFrame in descending order based on the challenges_won column
Q11 = Q11.sort_values(by=challenges_won_column, ascending=False)
# Step 4: Select the row with the contestant who has won the most challenges
Q11 = Q11.iloc[0:1, :]
print(Q11)
```
Make sure to replace `'challenges_won'` with the actual name of the column in your DataFrame that contains the number of challenges won by each contestant. This code will return a DataFrame that includes the row for the contestant who has won the most number of challenges, with the index and missing values remaining unchanged.